0. Annotation
1. Stereotype Annotation
Stereotype Annotation은 Bean을 등록할 때 사용할 수 있는 annotation이다.
Stereotype | 적용 대상 |
@Controller | MVC Controller에 사용 |
@Service | Service 계층 |
@Repository | DB에 접근하는 계층 ex) DAO |
@Component | 위의 계층에 속하지 않은 경우 ex) DTO 등 |
2. 의존 관계 설정 Annotation
Annotation | 설명 |
@Autowired | Spring에서만 사용 가능 멤버 변수, setter, constructor, 일반 method에 사용 가능하며 타입에 맞춰서 연결함 예시 => 2022.04.20 - [웹프로그래밍/Spring] - Spring (2) - IoC와 Container |
@Qualifer("name") | 같은 타입의 bean이 여러 개여서 Autowired한 객체들을 식별해야 할 때 |
1. Annotation 읽어서 객체(Bean)를 등록할 XML 파일 생성
앞서 본 "Container에 객체(Bean) 설정하기2 : XML"의 1번 과정과 동일하다.
이 글만 볼 경우를 위해 아래 더보기로 작성해놓았다.
src 우클릭 > [New] > [Spring Bean Configuration File]
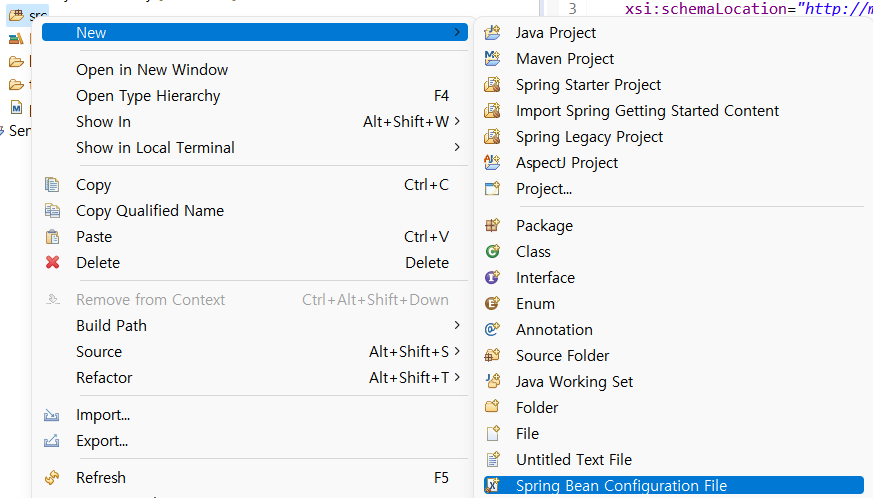
파일 이름은 보통 application.xml을 사용한다. [Next] > beans 선택 > [Finish]
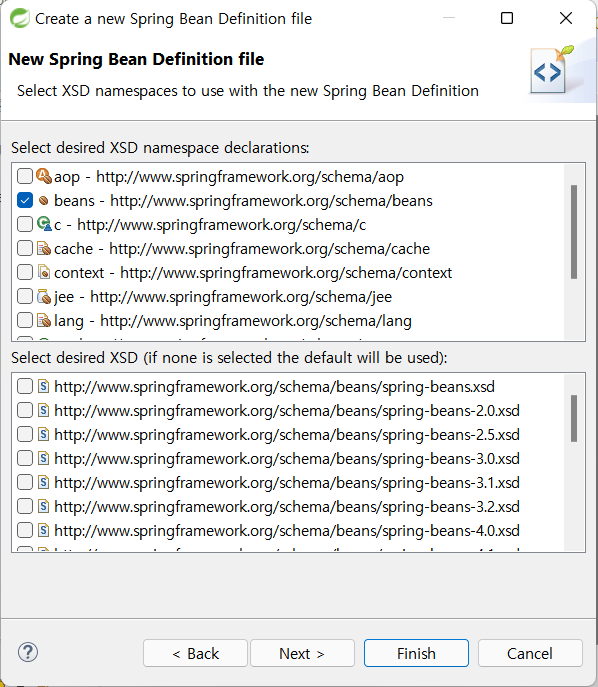
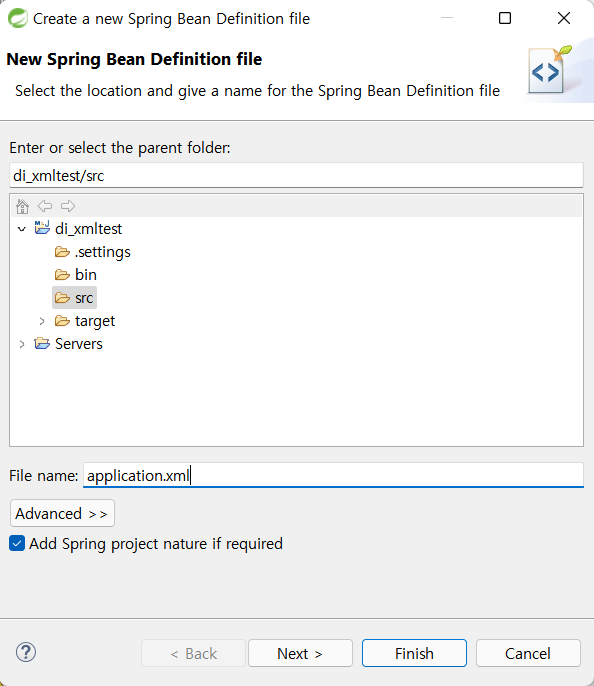
2. Annotation 작성하기
TestService.java, TestDaoImpl1, TestDaoImpl2에 annotation을 붙여준다.
Interface인 TestDao는 실제로 생성해야 할 객체가 아니므로 붙여주지 않는다.
1) TestService.java
@Autowired = 의존 관계 설정
@Qualifier("구현한 객체 이름") = 주입할 객체가 동일한 Interface를 구현해서 타입이 같을 때 구분하기 위해 사용
private TestDaoImpl1 td1;을 작성해도 같은 작업이 필요하다.
이유는 Container는 타입으로 객체를 구분해서, TestDao타입으로 TestDaoImpl1과 TestDaoImpl2로 2개가 있는데 어느 것을 주입해줘야 하는지 알려줘야 한다.
//TestService.java
package com.test.di1;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Service;
@Service("ts")
public class TestService {
@Autowired
@Qualifier("d1")
private TestDao td1; //private TestDaoImpl1 td1;을 해도 같은 작업 필요
@Autowired
@Qualifier("testDaoImpl2")//default는 맨 첫문자가 소문자인 클래스 이름
private TestDao td2;
public TestService() {
System.out.println("TestService 생성자!");
}
public void TestPrint() {
td1.testPrint();
td2.testPrint();
}
}
2) TestDaoImpl1 & TestDaoImpl2
Dao는 @Repository로 annotation을 붙이며, 이름을 지정할 수 있다.
//방법1
@Repository(value = "d1")
//방법2
@Repository("d1")
TestDaoImpl1에는 이름을 붙여주었고, TestDaoImpl2는 이름을 붙여주지 않았다. 이름을 지정하지 않으면 default는 클래스 이름에서 첫 문자가 소문자인 문자열이 된다. 즉, testDaoImpl2가 이름이다.
//TestDaoImpl1.java
import org.springframework.stereotype.Repository;
@Repository("d1")
public class TestDaoImpl1 implements TestDao { ... }
//TestDaoImpl2.java
import org.springframework.stereotype.Repository;
@Repository
public class TestDaoImpl2 implements TestDao { ... }
3. application.xml 설정 파일에 작성
먼저, [Namespaces] > [context]를 체크하고 Source로 돌아온다.
application.xml에 한 줄만 작성해주면 된다. base-package에 작성한 범위 안에 있는 @Annotation을 스캔해서 객체를 등록하겠다는 의미이다.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<context:component-scan base-package="com.test.di1"></context:component-scan>
</beans>
결과 Console창
ts1과 ts2의 hashcode가 같으므로 singleton으로 생성된 것도 확인할 수 있다.
'Programming > Spring' 카테고리의 다른 글
Spring (4) - MySQL과 연결하기 (0) | 2022.04.25 |
---|---|
Spring (3) - Container에 객체(Bean) 설정하기4 : Java (0) | 2022.04.23 |
Spring (3) - Container에 객체(Bean) 설정하기2 : XML (0) | 2022.04.22 |
Spring (3) - Container에 객체(Bean) 설정하기1 : 프로젝트 생성 (0) | 2022.04.22 |
Spring 기타(1) - 객체 간 결합도가 낮은 이유 (0) | 2022.04.20 |